Posts tagged as JavaScript
#JavaScript
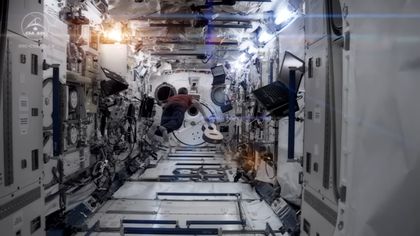
From Gatsby.js to Astro
How I migrated my website from Gatsby.js to Astro. What I liked about Astro and some problems I encountered during the migration.
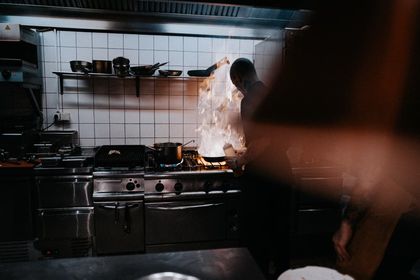
Using Notion as a CMS for Gatsby.js
How I use Notion as a CMS for my website built with Gatsby.js. The script can also be used with different static site generator like Astro and Next.js
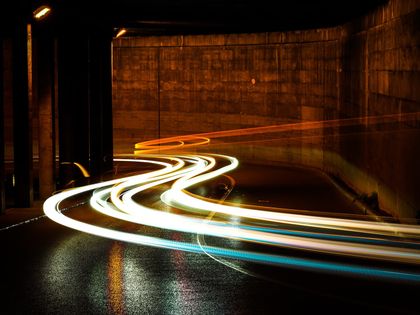
Improving Core Web Vitals of a React Application
A case study of changes implemented to improve the performances of a React application.
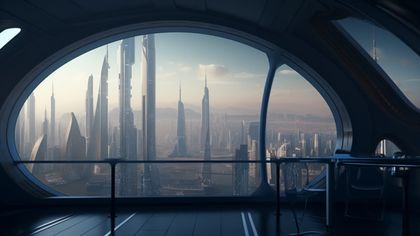
How to publish an npm package for ESM and CommonJS with TypeScript
A step by step tutorial on how to publish an npm package for ECMAScript Modules (ESM) and CommonJS (CJS) with TypeScript
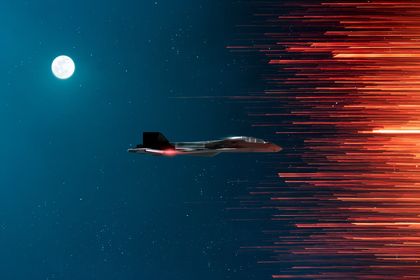
Turborepo: a Monorepo Revolution
My thoughts about Turborepo, a tool that facilitates your monorepo management.
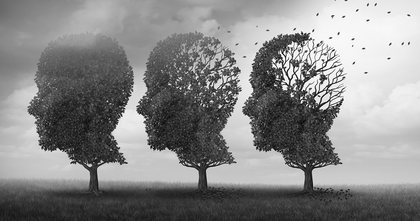
Vue.js Performance Improvement with Memoization
How I reduced the rendering of a complex component by 10 (from ~4s to ~0.3s).
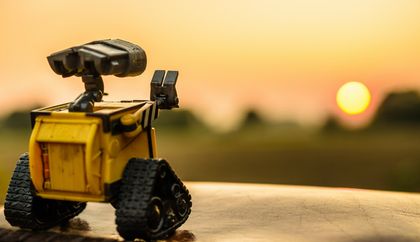
Vue.js Testing Made it Easy (with Testing Library)
How Testing Library helps you to write better integration test
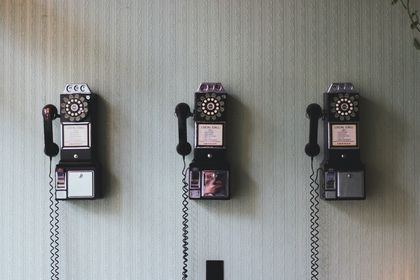
How to mock Axios HTTP calls with Jest
How to unit test components that have HTTP calls.
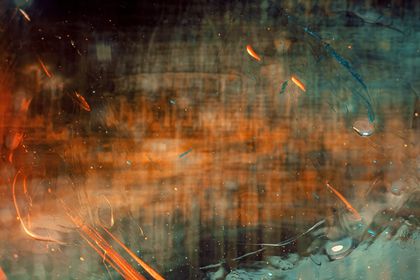
async/await without try...catch!
A cool wrapper to write promises without using a try...catch block
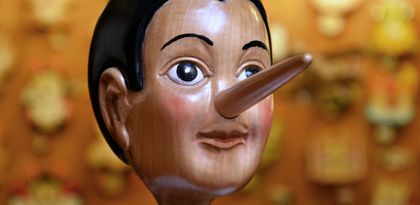
Why you shouldn't pay too much attention to your code coverage
High code coverage doesn't guarantee quality: You can write tests that cover every line but miss edge cases or core functionality.
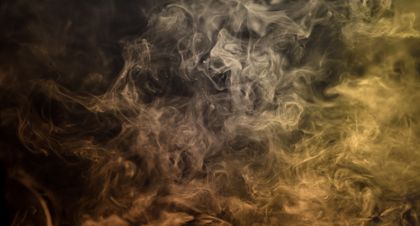
How to generate social share images with Gatsby
How I created dynamic Open Graph images for my Gatsby website
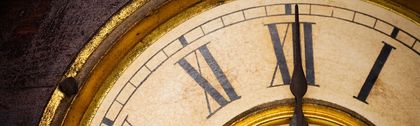
How to mock Date with Jest
A guide on how to deal with unit tests that uses the JavaScript Date object.
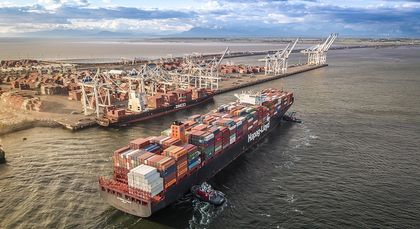
3 Tips for Scaling Large Vue.js Application
1. Split your application into completely isolated modules. 2. Consider micro-frontends architecture. 3. Don't put everything in the Vuex Store
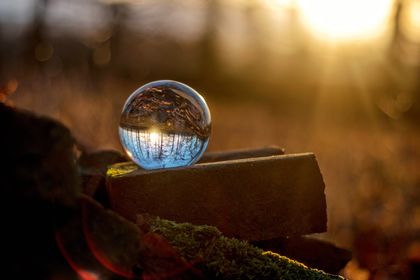
Hello GatsbyJS!
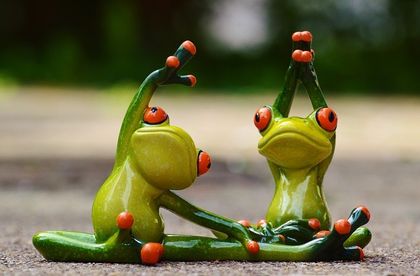
Typical JavaScript interview exercises (explained)
Level up your JavaScript interview skills! This post tackles common exercises, offering clear explanations to help you ace the coding challenge.
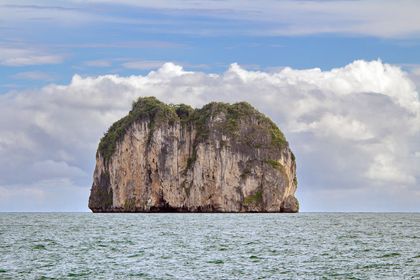
Immutability in JavaScript (without library)
A deep dive into JS immutability, guiding you on how to achieve it without relying on external libraries.
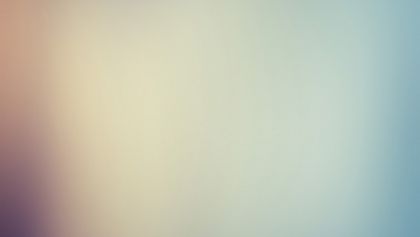
Chaining Javascript filters recursively
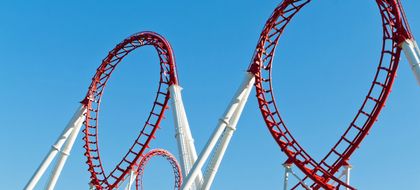
No more for/while loop in JavaScript
Some alternatives to traditional for/while loops in JavaScript. Also how to address set theory with array of objects (union, intersection and difference).