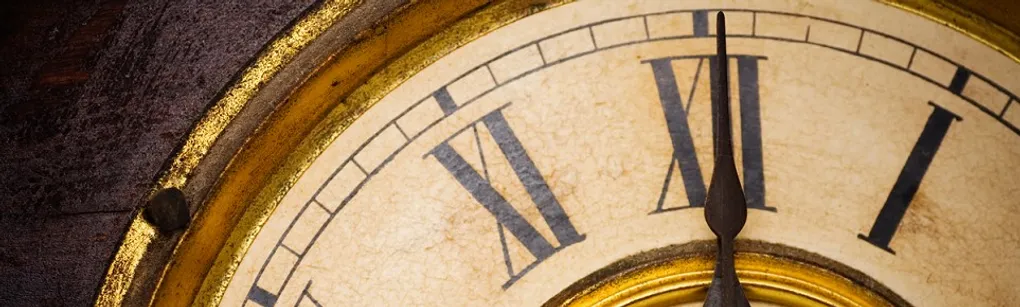
I spent too many hours trying to mock correctly the JavaScript’s Date object. I tried a few things I’ve found on Google… without success. I finally ended up with a kinda handcrafted solution.
Every time we mock, we diverge from the real world scenario.
For this reason, I tend not to mock… but sometimes, there are not many choices. Fortunately, Date is one good exception!
Everybody on the same timezone!
To prevent problems related to timezones (e.g. date formating), you can set node timezone in jest config file. Now you are sure all tests are executed on the same timezone no matter where your colleagues or your CI server are.
process.env.TZ = 'GMT'
module.exports = { // ...}
See also: the full list of timezones (column TZ database name)
Mock Date.now
Let’s say I want to test a dashboard component which tells me “hello” with the date of the day. The lazy way is to only test the Hello part (without the date). Because it changes every day. But you won’t test the date formatting part.
If you want to, you gonna have to mock Date.now()
and put a default one.
const RealNow = Date.now
beforeAll(() => { global.Date.now = jest.fn(() => new Date('2019-04-07T10:20:30Z').getTime())})
afterAll(() => { global.Date.now = RealNow})
Now in the same file, you can add something like the following:
it('should show a formatted date of today', async () => { const dashboard = await Mount(<Dashboard />) expect(dashboard).toHaveText('Hi Max, today is 7 April 2019')})
💡RealDate
store “real” Date instance, so we can put it back afterwards.
Using Moment.js?
You are probably using the very popular moment.js library. If so, mocking Date.now
, will probably
not be enough. A workaround is to
mock the entire node module.
// <root>/__mocks__/moment.jsconst moment = jest.requireActual('moment')
Date.now = () => new Date('2019-04-07T10:20:30Z').getTime()
module.exports = moment
With this solution, you don’t need beforeAll()
/afterAll()
listener. This mock will be effective
for all tests. And, every time moment()
is called, return date will be the same 🎉
Big shout out to Avalander for pointing out some errors. https://dev.to/avalander/comment/ibc7
About the author
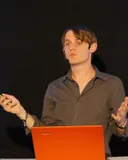
Hey, I'm Maxence Poutord, a passionate software engineer. In my day-to-day job, I'm working as a senior front-end engineer at Orderfox. When I'm not working, you can find me travelling the world or cooking.
Follow me on Bluesky